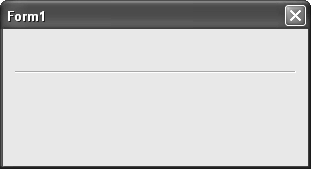
[C#]
// separator bevel line label1.AutoSize = false; label1.Height = 2; label1.BorderStyle = BorderStyle.Fixed3D;
source : http://www.csharp-examples.net/separator-line/
// separator bevel line label1.AutoSize = false; label1.Height = 2; label1.BorderStyle = BorderStyle.Fixed3D;
source : http://www.csharp-examples.net/separator-line/
nvarchar
and integer
column. The nvarchar
column will not allow null
s and the integer will allow null
s. The schema creations script for the same is:--This is the database table
CREATE TABLE dbo.SampleTable
(
Id int NOT NULL IDENTITY (1, 1),
SampleString nvarchar(64) NOT NULL,
SampleInt int NULL
) ON [PRIMARY]
-- Create a table data type
CREATE TYPE [dbo].[SampleDataType] As Table
(
--This type has structure similar to the DB table
SampleString Nvarchar(64) Not Null -- Having one String
, SampleInt Int -- and one int
)
SampleDataType
type as input parameter. Inside the stored procedure, the parameter is available as a temporary table. This table however has to be readonly.--This is the Stored Procedure
CREATE PROCEDURE [dbo].[SampleProcedure]
(
-- which accepts one table value parameter.
-- It should be noted that the parameter is readonly
@Sample As [dbo].[SampleDataType] Readonly
)
AS
Begin
-- We simply insert values into the DB table from the parameter
-- The table value parameter can be used like a table with only read rights
Insert Into SampleTable(SampleString,SampleInt)
Select SampleString, SampleInt From @Sample
End
-- This is the sample script to test the SP
-- An instance of the Table parameter type is created
Declare @SampelData As [dbo].[SampleDataType]
-- and then filled with the set of values
Insert Into @SampelData(SampleString, SampleInt) Values('1',1);
Insert Into @SampelData(SampleString, SampleInt) Values('2',null);
Insert Into @SampelData(SampleString, SampleInt) Values('3',3);
Select * From @SampelData
-- we then call the SP to store the values
Exec SampleProcedure @SampelData
Select * From SampleTable
nvarchar
is represented as string
on the C# end.DataTable
,IEnumerable
, Linq object, Data reader, etc. In this article, we will focus on the DataTable
. All we do is create aDataTable
, define columns parallel to our Table data type and fill them up.//To represent the table parameter in C#, we need to either
//have a set of entities which are IEnumreable
//or a data reader or a Data table.
//In this example we create a data table with same name as the type we have in the DB
DataTable dataTable = new DataTable("SampleDataType");
//we create column names as per the type in DB
dataTable.Columns.Add("SampleString", typeof(string));
dataTable.Columns.Add("SampleInt", typeof(Int32));
//and fill in some values
dataTable.Rows.Add("99", 99);
dataTable.Rows.Add("98", null);
dataTable.Rows.Add("97", 99);
SqlParameter
. The type of this parameter is Structured
. The details are as shown in the code snippet. The other code to call the SP is trivial and may be seen in the given code.SqlParameter parameter = new SqlParameter();
//The parameter for the SP must be of SqlDbType.Structured
parameter.ParameterName="@Sample";
parameter.SqlDbType = System.Data.SqlDbType.Structured;
parameter.Value = dataTable;
command.Parameters.Add(parameter);
CREATE TYPE dbo.MyDataTable -- you can be more speciifc here
AS TABLE
(
col1 INT,
col2 DATETIME
-- etc etc. The columns you have in your data table.
);
GO
CREATE PROCEDURE dbo.InsertMyDataTable
@dt AS dbo.MyDataTable READONLY
AS
BEGIN
SET NOCOUNT ON;
INSERT dbo.RealTable(column list) SELECT column list FROM @dt;
END
GO
DataTable tvp = new DataTable();
// define / populate DataTable
using (connectionObject)
{
SqlCommand cmd = new SqlCommand("dbo.InsertMyDataTable", connectionObject);
cmd.CommandType = CommandType.StoredProcedure;
SqlParameter tvparam = cmd.Parameters.AddWithValue("@dt", tvp);
tvparam.SqlDbType = SqlDbType.Structured;
cmd.ExecuteNonQuery();
}
public abstract class Shape { public abstract float calArea(); } |
public class Circle extends Shape { double radius; public Circle(double radius) { this.radius = radius; } @Override public double calArea() { return Math.PI * Math.pow(radius, 2); } } |
public class Rectangle extends Shape { double lenght; double height; public Rectangle(double lenght, double height) { this.lenght = lenght; this.height = height; } @Override public double calArea() { return lenght * height; } } |
public class Triangle extends Shape { double base; double height; public Triangle(double base, double height) { this.base = base; this.height = height; } @Override public double calArea() { return base * height * 0.5; } } |
public class MainClass { public static void main(String[] args) { Circle c = new Circle(5); Triangle t = new Triangle(3, 6); Rectangle r = new Rectangle(10, 5); System.out.println(c.calArea()); System.out.println(t.calArea()); System.out.println(r.calArea()); } } |
public class MainClass { public static void main(String[] args) { Shape[] s = {new Circle(5), new Triangle(3, 6), new Rectangle(10, 5)}; for(int i = 0; i < s.length; i++) System.out.println(s[i].calArea()); } } |
public class MainClass { private static void printShape(Shape shape) { System.out.println(shape.calArea()); } public static void main(String[] args) { Circle c = new Circle(5); Triangle t = new Triangle(3, 6); Rectangle r = new Rectangle(10, 5); printShape(c); printShape(t); printShape(r); } } |
public interface IFlyAble { public void Fly(); } public interface IRunAble { public void Run(); } |
public class Human implements IRunAble { @Override public void Run() { System.out.println("Human Run"); } } |
public class Bird implements IFlyAble{ @Override public void Fly() { System.out.println("Bird Fly"); } } |
public class Plane implements IFlyAble, IRunAble{ @Override public void Run() { System.out.println("Plane Run"); } @Override public void Fly() { System.out.println("Plane Fly"); } } |
private static void goFly(IFlyAble flyAble) { flyAble.Fly(); } private static void goRun(IRunAble runAble) { runAble.Run(); } |
public static void main(String[] args) { Human h = new Human(); Bird b = new Bird(); Plane p = new Plane(); goRun(h); goFly(b); goRun(p); goFly(p); } |